Understanding RESTful APIs: Best Practices and Examples
Dev
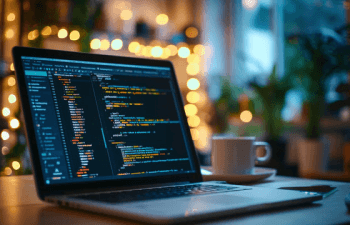
In the modern era of software development, RESTful APIs (Application Programming Interfaces) have revolutionized the way applications communicate over the internet. RESTful APIs adhere to the principles of Representational State Transfer (REST), an architectural style that enables seamless interaction between different software systems. This architectural approach has had a significant impact on web development, providing a standardized way for applications to interact, share data, and integrate functionalities. RESTful APIs are widely used due to their simplicity, scalability, and flexibility, making them a cornerstone of modern web development.
What is a RESTful API?
A RESTful API is an application programming interface that adheres to the principles of Representational State Transfer (REST) architecture. REST is an architectural style that uses standard HTTP methods and is designed around the concept of resources, which can be identified by URLs. Each resource can be accessed and manipulated using a stateless protocol, typically HTTP.
Key Principles of RESTful APIs
- Statelessness: Each request from a client to a server must contain all the information needed to understand and process the request. The server does not store any client context between requests.
- Client-Server Architecture: The client and server operate independently, allowing for scalable and flexible applications.
- Uniform Interface: The API should have a consistent, uniform interface, simplifying interactions for developers.
- Resource-Based: Resources (such as data objects or services) are identified by URLs, and the representation of these resources is transferred over the network.
- HTTP Methods: RESTful APIs use standard HTTP methods like GET, POST, PUT, DELETE, PATCH, etc., to perform CRUD (Create, Read, Update, Delete) operations on resources.
- Stateless Communication: Each request from client to server must contain all the information the server needs to fulfill that request.
Best Practices for RESTful API Design
1. Use Nouns to Represent Resources
Resources should be represented using nouns, not verbs. For example, if you are creating an API for a bookstore, the endpoint for books should be `/books`
rather than `/getBooks`
or `/createBook`
.
2. Use HTTP Methods Appropriately
Leverage the appropriate HTTP methods for different types of operations:
- GET: Retrieve a resource or a collection of resources.
- POST: Create a new resource.
- PUT: Update an existing resource.
- DELETE: Remove a resource.
- PATCH: Apply partial updates to a resource.
3. Use Plural Nouns
Use plural nouns for resource names to keep the API consistent and intuitive. For example, use `/books`
rather than `/book`
.
4. Use Clear and Consistent Naming Conventions
Ensure that your API endpoints are clear and consistent. This makes the API more intuitive and easier to use. For example, use kebab-case or snake_case consistently throughout your API.
5. Provide Meaningful HTTP Status Codes
Return appropriate HTTP status codes to indicate the outcome of an API request:
- 200 OK: The request was successful.
- 201 Created: A resource was successfully created.
- 204 No Content: The request was successful, but there is no representation to return (e.g., after a DELETE request).
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication is required and has failed or has not been provided.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server.
6. Use Pagination for Large Data Sets
For endpoints that return a large number of resources, implement pagination to improve performance and user experience. Include metadata such as total count, limit, offset, and links to next and previous pages.
7. Implement Filtering, Sorting, and Searching
Allow clients to filter, sort, and search resources to retrieve only the data they need. Use query parameters to implement these features. For example, `/books?author=JohnDoe&sort=title`
.
8. Provide Detailed Error Messages
When an error occurs, return detailed and informative error messages. Include information about what went wrong and how to fix it. This helps developers troubleshoot issues more efficiently.
9. Secure Your API
Implement security best practices to protect your API. Use HTTPS to encrypt data, require authentication (e.g., OAuth, API keys), and validate and sanitize inputs to prevent attacks such as SQL injection and cross-site scripting (XSS).
10. Version Your API
Version your API to manage changes and ensure backward compatibility. Include the version number in the URL, such as `/v1/books`
or in the request header.
Real-World Examples of RESTful APIs
To better understand how RESTful APIs are used in real-world applications, let's look at some examples from popular services.
Example 1: GitHub API
GitHub provides a RESTful API that allows developers to interact with their repositories, issues, pull requests, and more. Here are some key endpoints:
- GET /repos/{owner}/{repo}: Retrieve information about a specific repository.
- POST /repos/{owner}/{repo}/issues: Create a new issue in a repository.
- PATCH /repos/{owner}/{repo}/issues/{issue_number}: Update an existing issue.
Example 2: Twitter API
Twitter's RESTful API allows developers to access and interact with Twitter data, such as tweets, user profiles, and trends. Key endpoints include:
- GET /statuses/user_timeline: Retrieve a collection of the most recent tweets posted by the specified user.
- POST /statuses/update: Post a new tweet.
- DELETE /statuses/destroy/{id}: Delete a tweet.
Example 3: Google Maps API
Google Maps provides a RESTful API for accessing various mapping services, including geocoding, directions, and places. Key endpoints include:
- GET /maps/api/geocode/json: Get geocoding data for a given address.
- GET /maps/api/directions/json: Get directions between two locations.
- GET /maps/api/place/nearbysearch/json: Search for places near a given location.
Implementing a Simple RESTful API
To illustrate how to implement a RESTful API, let's create a simple API for managing a library of books. We'll use Node.js and Express.js for this example.
Setting Up the Project
1. Initialize the Project:
mkdir library-api
cd library-api
npm init -y
2. Install Dependencies:
npm install express body-parser
3. Create the Main Application File:
Create a file named `app.js`
and add the following code:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
const PORT = process.env.PORT || 3000;
app.use(bodyParser.json());
const books = [];
// Routes
app.get('/books', (req, res) => {
res.json(books);
});
app.post('/books', (req, res) => {
const book = req.body;
books.push(book);
res.status(201).json(book);
});
app.get('/books/:id', (req, res) => {
const book = books.find(b => b.id === req.params.id);
if (book) {
res.json(book);
} else {
res.status(404).send('Book not found');
}
});
app.put('/books/:id', (req, res) => {
const index = books.findIndex(b => b.id === req.params.id);
if (index !== -1) {
books[index] = req.body;
res.json(books[index]);
} else {
res.status(404).send('Book not found');
}
});
app.delete('/books/:id', (req, res) => {
const index = books.findIndex(b => b.id === req.params.id);
if (index !== -1) {
books.splice(index, 1);
res.status(204).send();
} else {
res.status(404).send('Book not found');
}
});
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Testing the API
To test the API, you can use tools like Postman or curl to make HTTP requests to the endpoints.
1. GET /books:
curl -X GET http://localhost:3000/books
2. POST /books:
curl -X POST http://localhost:3000/books -H "Content-Type: application/json" -d '{"id": "1", "title": "1984", "author": "George Orwell"}'
3. GET /books/1:
curl -X GET http://localhost:3000/books/1
4. PUT /books/1:
curl -X PUT http://localhost:3000/books/1 -H "Content-Type: application/json" -d '{"id": "1", "title": "Animal Farm", "author": "George Orwell"}'
5. DELETE /books/1:
curl -X DELETE http://localhost:3000/books/1
Conclusion
Understanding and implementing RESTful APIs is crucial for modern web development. By following best practices, you can create APIs that are efficient, scalable, and easy to use. Whether you're integrating third-party services or building your own applications, RESTful APIs provide a powerful and flexible way to connect and interact with different software components. With the principles and examples provided in this blog post, you're well-equipped to design and use RESTful APIs effectively.